[SOLVED] Fixing the ‘Bash: Bad Substitution’ Error
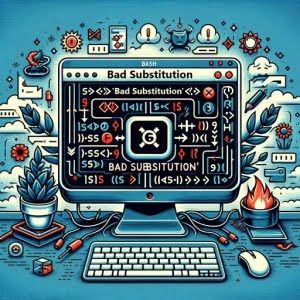
Ever found yourself stuck with a ‘bash: bad substitution’ error? Like a mischievous puzzle, Bash can sometimes throw curveballs at you. Many developers find themselves puzzled when they encounter this error, but don’t worry, we’re here to help.
Think of Bash scripting as a powerful tool, allowing us to automate tasks and manipulate data. However, it can be a bit tricky, especially when dealing with variable substitutions. This is where the ‘bash: bad substitution’ error often comes into play.
In this guide, we’ll walk you through the process of solving the ‘bash: bad substitution’ error, from the basics to more advanced techniques. We’ll cover everything from understanding the cause of the error, how to fix it using basic Bash syntax, to discussing more complex scripts and alternative approaches.
So, let’s dive in and start mastering Bash scripting!
TL;DR: How Do I Fix the ‘Bash: Bad Substitution’ Error?
The
'bash: bad substitution'
error usually occurs when you’re trying to use a variable in a way that Bash doesn’t understand. For example, usingecho ${var[0]}
, instead ofecho ${var:0:1}
, to access specific characters of a string will result in abad substitution
error.
Here’s a simple example of what not to do:
var=Hello
echo ${var[0]}
# Output:
# bash: bad substitution
In this example, we’re trying to access the first character of the string ‘Hello’ stored in the variable var
using an array-like syntax (${var[0]}
), which Bash doesn’t understand, resulting in the ‘bash: bad substitution’ error.
Instead, use parameter expansion like this:
var=Hello
echo ${var:0:1}
# Output:
# H
In this corrected example, we’re using Bash’s parameter expansion syntax to access the first character of the string. The ${var:0:1}
syntax tells Bash to return a substring of var
starting at index 0 and of length 1, effectively giving us the first character.
This is just a basic way to avoid the ‘bash: bad substitution’ error, but there’s much more to learn about Bash scripting and variable manipulation. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding the ‘Bash: Bad Substitution’ Error
- Correct Use of Parameter Expansion
- Delving Deeper: Advanced Bash Scripting
- Exploring Alternatives: Beyond Basic Bash
- Troubleshooting ‘Bash: Bad Substitution’ and Other Common Issues
- Bash Scripting, Variables, and Parameter Expansion
- The Relevance of Bash Scripting in Today’s Tech Landscape
- Wrapping Up: The ‘Bash: Bad Substitution’ Error
Understanding the ‘Bash: Bad Substitution’ Error
The ‘bash: bad substitution’ error is a common issue that developers encounter when dealing with Bash scripts. It occurs when Bash cannot understand the syntax used, especially when dealing with variable substitutions.
Let’s take a look at a common scenario that leads to this error:
var=Hello
# Trying to access the first character of the string using array-like syntax
echo ${var[0]}
# Output:
# bash: bad substitution
In this example, we’re trying to access the first character of the string ‘Hello’ stored in the variable var
using an array-like syntax (${var[0]}
). Bash doesn’t understand this syntax when applied to a string, resulting in the ‘bash: bad substitution’ error.
So how do we fix this? The solution lies in understanding and using parameter expansion correctly.
Correct Use of Parameter Expansion
Parameter expansion is a powerful feature in Bash that allows us to manipulate strings in various ways. One of its uses is to extract substrings from a string.
Here’s how you can use parameter expansion to avoid the ‘bash: bad substitution’ error:
var=Hello
# Using parameter expansion to access the first character of the string
echo ${var:0:1}
# Output:
# H
In this corrected example, we’re using Bash’s parameter expansion syntax to access the first character of the string. The ${var:0:1}
syntax tells Bash to return a substring of var
starting at index 0 and of length 1, effectively giving us the first character.
This is a basic yet powerful way to avoid the ‘bash: bad substitution’ error. Understanding and correctly using parameter expansion can greatly enhance your Bash scripting skills and open up a whole new world of possibilities.
Delving Deeper: Advanced Bash Scripting
As we progress in our Bash scripting journey, we will undoubtedly encounter more complex scripts. The ‘bash: bad substitution’ error can become more common as we start manipulating variables in more advanced ways. Let’s explore an intermediate-level scenario and see how to avoid this error.
Using Arrays in Bash
Bash supports arrays, and the syntax we used in the previous examples (${var[0]}
) would be perfectly valid if var
was an array. Here’s an example:
var=('Hello' 'World')
# Accessing the first element of the array
echo ${var[0]}
# Output:
# Hello
In this example, var
is an array containing two elements: ‘Hello’ and ‘World’. The ${var[0]}
syntax is used to access the first element of the array, which works as expected.
Substring Extraction with Arrays
But what if we want to extract a substring from an array element, similar to what we did with the string in the previous section? Here’s how to do it:
var=('Hello' 'World')
# Using parameter expansion to access the first character of the first element
echo ${var[0]:0:1}
# Output:
# H
In this example, we’re using parameter expansion with an array. The ${var[0]:0:1}
syntax tells Bash to return a substring of the first element of var
(which is ‘Hello’), starting at index 0 and of length 1, effectively giving us the first character.
This is a more advanced use case that demonstrates the flexibility of Bash scripting and parameter expansion. By understanding these concepts and using them correctly, you can avoid the ‘bash: bad substitution’ error even in more complex scripts.
Exploring Alternatives: Beyond Basic Bash
Bash scripting is incredibly versatile, and there are often multiple ways to achieve the same result. If you’re looking for alternative approaches to avoid the ‘bash: bad substitution’ error, you’re in the right place. Let’s explore some additional methods and tools that you can use.
Using AWK for String Manipulation
AWK is a powerful text processing language that’s available on most Unix-like systems. It’s particularly good at handling strings, making it a useful tool to avoid the ‘bash: bad substitution’ error.
Here’s an example of how you can use AWK to extract the first character of a string:
var=Hello
# Using AWK to get the first character of the string
echo $var | awk '{print substr($0, 1, 1)}'
# Output:
# H
In this example, we’re piping the value of var
into AWK, which uses the substr
function to return a substring starting at the first character and of length 1.
Using Cut Command
The cut command is another useful tool for manipulating strings. It can be used to extract sections from each line of files. Here’s how to use cut to get the first character of a string:
var=Hello
# Using cut to get the first character of the string
echo $var | cut -c1
# Output:
# H
In this example, we’re piping the value of var
into cut, which extracts the first character of the string.
Each of these methods comes with its own advantages and disadvantages. While AWK and cut can be more powerful and flexible than Bash’s built-in parameter expansion, they also add an extra layer of complexity to your scripts. It’s important to choose the right tool for the job, considering your specific needs and the complexity of your scripts.
Troubleshooting ‘Bash: Bad Substitution’ and Other Common Issues
Bash scripting, like any other programming or scripting language, can sometimes be a source of frustration. The ‘bash: bad substitution’ error is one of the common issues that developers encounter. However, with a little bit of knowledge and some troubleshooting skills, these issues can be resolved easily.
Variable Name Typo
One common mistake is a typo in the variable name. This can lead to unexpected errors. For example:
var=Hello
# Typo in the variable name
echo ${vr:0:1}
# Output:
# bash: vr: bad substitution
In this example, we made a typo in the variable name (vr
instead of var
). Bash doesn’t recognize vr
as a variable, leading to the ‘bash: bad substitution’ error.
Unset Variables
Another common issue is trying to use an unset variable. If a variable hasn’t been set, Bash will treat it as a null string:
# Unset variable
echo ${var:0:1}
# Output:
#
In this example, we’re trying to use parameter expansion on an unset variable (var
). Since var
hasn’t been set, Bash treats it as a null string, and the output is an empty line.
Incorrect Parameter Expansion Syntax
Using incorrect syntax for parameter expansion is a common cause of the ‘bash: bad substitution’ error. It’s important to remember the correct syntax: ${var:start:length}
.
var=Hello
# Incorrect syntax for parameter expansion
echo ${var:0,1}
# Output:
# bash: var:0,1: bad substitution
In this example, we’re using a comma (,
) instead of a colon (:
) in the parameter expansion syntax. This leads to the ‘bash: bad substitution’ error because Bash doesn’t recognize this syntax.
Remember, troubleshooting is a key skill in any form of programming or scripting. Understanding common issues and their solutions can save you a lot of time and frustration.
Bash Scripting, Variables, and Parameter Expansion
To fully grasp the ‘bash: bad substitution’ error, it’s important to understand the fundamentals of Bash scripting, variables, and parameter expansion.
Bash Scripting Basics
Bash (Bourne Again SHell) is a popular command-line interpreter or shell. It allows users to interact with the operating system by typing commands. Bash scripting takes this a step further by allowing us to automate these commands.
A Bash script is a plain text file containing a series of commands. These scripts can run complex and repetitive tasks automatically, making them a powerful tool for system administrators and developers.
Understanding Variables in Bash
In Bash scripting, a variable is a symbol or name that stands for a value. Variables are used to store information and can be used anywhere that a literal value can.
Here’s an example of a variable in a Bash script:
# Define a variable
greeting='Hello, world!'
# Use the variable
echo $greeting
# Output:
# Hello, world!
In this example, greeting
is a variable that stores the string ‘Hello, world!’. We can then use this variable in our script by referring to it with a dollar sign ($
).
Parameter Expansion: The Power Tool in Bash
Parameter expansion is a mechanism in Bash that allows us to manipulate and use the value stored in a parameter (like a variable) in various ways.
One common use of parameter expansion is to extract a substring from a string. The syntax for this is ${variable:start:length}
, where start
is the starting index (0-based) and length
is the length of the substring.
Here’s an example:
# Define a variable
name='Alice'
# Use parameter expansion to get the first two characters
echo ${name:0:2}
# Output:
# Al
In this example, ${name:0:2}
uses parameter expansion to get a substring of name
starting at index 0 and of length 2. The output is ‘Al’, the first two characters of ‘Alice’.
Understanding Bash scripting, variables, and parameter expansion is key to mastering Bash and avoiding common errors like ‘bash: bad substitution’.
The Relevance of Bash Scripting in Today’s Tech Landscape
Bash scripting is not just a tool of the past; it’s a relevant and powerful tool used widely in today’s tech landscape. It’s especially prevalent in system administration and automation tasks.
Bash Scripting for System Administration
System administrators often use Bash scripts to automate routine tasks. From managing users and updating systems to monitoring system health, Bash scripting can make these tasks more efficient.
# Sample Bash script to monitor disk usage
df -h | awk '$5 > "80%" {print "Disk usage alert: " $5 " used on " $1}'
# Output:
# Disk usage alert: 85% used on /dev/sda1
In this example, the Bash script uses the df
command to check disk usage and awk
to filter and format the output. If any disk usage exceeds 80%, it prints a warning message.
Automating Tasks with Bash Scripts
Automation is another area where Bash scripting shines. Developers can write scripts to automate tasks like deploying software, managing configurations, or processing logs.
# Sample Bash script to automate software deployment
# Pull the latest code
git pull origin main
# Build the software
make all
# Deploy the software
sudo make install
# Output:
# [Expected output from each command]
This example script automates the process of pulling the latest code from a Git repository, building the software using make
, and deploying it.
Further Learning: Shell Scripting and Command Line Interfaces
If you’re interested in Bash scripting, you might also want to explore related concepts like shell scripting and command line interfaces (CLIs). Shell scripting is similar to Bash scripting but can be used with other shells like Zsh or Fish. CLIs, on the other hand, are interfaces that allow users to interact with a system using text-based commands.
Further Resources for Bash Scripting Mastery
For those wanting to delve deeper into Bash scripting, here are some resources to explore:
- Devhints.io Bash Cheat Sheet: This cheat sheet provides a concise reference guide for various Bash commands and syntax.
Bash Scripting Tutorial: A comprehensive tutorial on Bash scripting, covering everything from basic to advanced topics.
- Advanced Bash-Scripting Guide: An in-depth guide to Bash scripting, suitable for those wanting to explore more advanced topics.
Wrapping Up: The ‘Bash: Bad Substitution’ Error
In this comprehensive guide, we’ve delved deep into the ‘bash: bad substitution’ error, exploring its causes, solutions, and alternative approaches. We’ve demystified this common pitfall in Bash scripting, turning it from a source of frustration into an opportunity for learning and growth.
We began with the basics, understanding the error and its most common cause: misuse of Bash’s variable substitution syntax. We examined how to correct this through proper use of parameter expansion, providing clear, practical examples along the way.
From there, we ventured into more advanced territory, discussing arrays in Bash and how they interact with variable substitution. We also explored alternative methods for string manipulation, such as AWK and the cut command, demonstrating the flexibility and power of Bash scripting.
Throughout our journey, we tackled common issues that you might encounter when dealing with Bash scripts, such as variable name typos, unset variables, and incorrect parameter expansion syntax. For each issue, we provided solutions and tips, equipping you with the knowledge to troubleshoot these problems effectively.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Parameter Expansion | Built into Bash, efficient | Requires understanding of syntax |
AWK | Powerful, flexible | Adds complexity to scripts |
Cut Command | Simple to use | Limited functionality |
Whether you’re a beginner just starting out with Bash scripting or an experienced developer looking to level up your skills, we hope this guide has given you a deeper understanding of the ‘bash: bad substitution’ error and how to avoid it.
Bash scripting is a powerful tool, and mastering it can open up a world of possibilities. With the knowledge and skills you’ve gained from this guide, you’re well equipped to tackle the ‘bash: bad substitution’ error and any other challenges that come your way. Happy scripting!