It’s not related to today’s topic but I’m sick with back pain. I hope I will get well soon..
Somedays ago I wrote post about qiskit. I think qiskit is very interesting package for quantum computing. And I stared to learn qiskit and quantum computer.
One of the interesting point of quantum computer is that it uses qubit instead of bit and the qubit can have an entangle state which means 0 or 1.
Today I made very simple quantum accumulator with qiskit.
Following code uses 4 qubit and 4 classical register.
Classical register used for results measurement.
And 4 kinds of gates.
Pauli X gate: x, this gate change |0> to |1>
hadamard gate: h, this gate generates entangle state |0> => 1/sq(2) [|0> + |1>]
control not gate: cx, this gate is same as NOT gate of classical computer
And gate: ccx, this gate is same ans AND gate.
Image of quantum circuit for 1 + 0 is below. First two qubits are used for input and third and forth qubits are used for output.
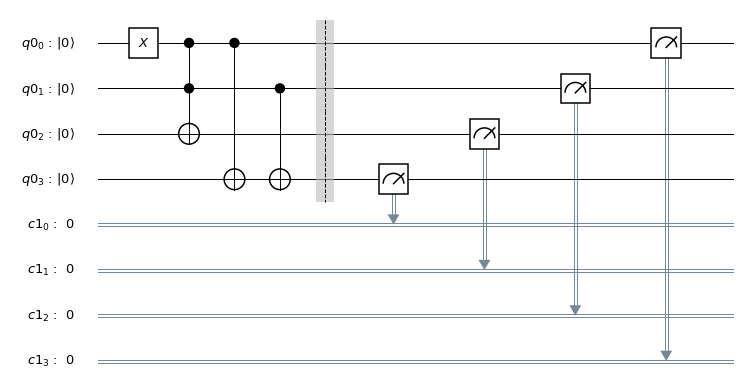
The code is below.
%matplotlib inline import numpy as np from qiskit import QuantumCircuit from qiskit import QuantumRegister from qiskit import execute from qiskit import BasicAer from qiskit import ClassicalRegister from qiskit.visualization import plot_histogram # I used simulator instead of real quantum computer backend_sim = BasicAer.get_backend('qasm_simulator') # 1 + 0 = 1 # Made 4 qubits and 4 classical bits q = QuantumRegister(4) c = ClassicalRegister(4) # make quantum circuit object and add gates. qc = QuantumCircuit(q,c) qc.x(q[0]) qc.ccx(q[0],q[1],q[2]) qc.cx(q[0],q[3]) qc.cx(q[1],q[3]) qc.barrier(q) qc.measure(q[3],c[0]) qc.measure(q[2],c[1]) qc.measure(q[1],c[2]) qc.measure(q[0],c[3]) qc.draw(output='mpl') # draw the circuit above.
OK let’s run the calculation.
# shots means number of trials because results of quantum computing depends on probability. job = execute(qc, backend_sim, shots=80) result = job.result() plot_histogram(result.get_counts(qc))
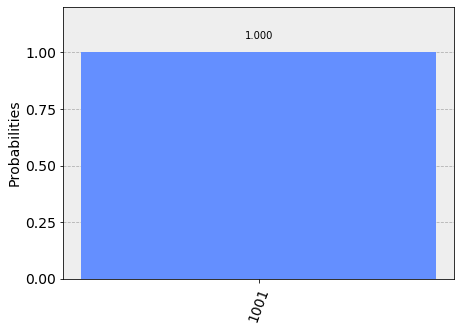
1001 meants qubit1(1) + qubit(0) = 01 with 100% probability! It seems work well.
Next, how about 1 + 1? I added additional Pauli X gate to q[1].
# 1 + 1 = 2 q = QuantumRegister(4) c = ClassicalRegister(4) c = ClassicalRegister(4) qc = QuantumCircuit(q,c) qc.x(q[0]) qc.x(q[1]) qc.ccx(q[0],q[1],q[2]) # and gate count up when q[0] and q[1] is 1 qc.cx(q[0],q[3]) qc.cx(q[1],q[3]) qc.barrier(q) qc.measure(q[3],c[0]) qc.measure(q[2],c[1]) qc.measure(q[1],c[2]) qc.measure(q[0],c[3]) qc.draw(output='mpl')
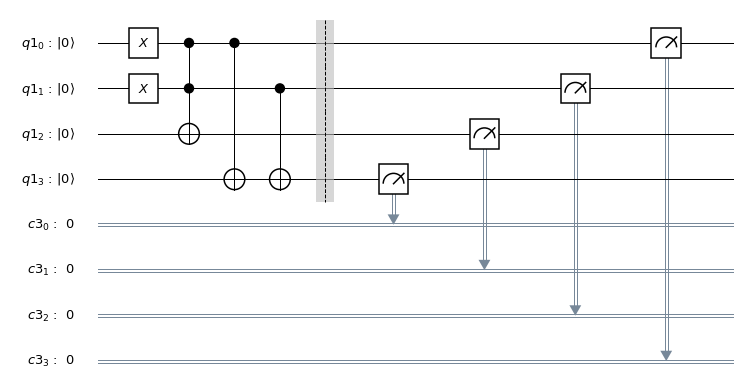
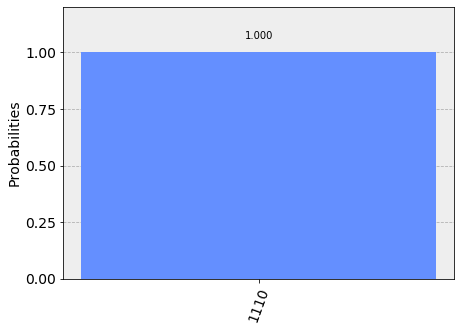
The results 1110 with 100% probability. It means qubit(1) + qubit(1) = 10, Binary 10 means 2 in decimal.
At last, write code with entangle state.
# 0/1 + 0/1 = 0, 1, 2 q = QuantumRegister(4) c = ClassicalRegister(4) c = ClassicalRegister(4) qc = QuantumCircuit(q,c) #qc.x(q[0]) #qc.x(q[1]) qc.h(q[0]) qc.h(q[1]) qc.ccx(q[0],q[1],q[2]) qc.cx(q[0],q[3]) qc.cx(q[1],q[3]) qc.barrier(q) qc.measure(q[3],c[0]) qc.measure(q[2],c[1]) qc.measure(q[1],c[2]) qc.measure(q[0],c[3]) qc.draw(output='mpl')
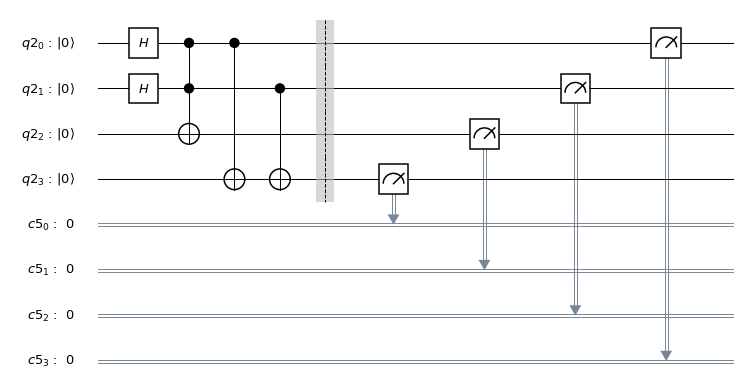
job = execute(qc, backend_sim, shots=80) result = job.result() plot_histogram(result.get_counts(qc))
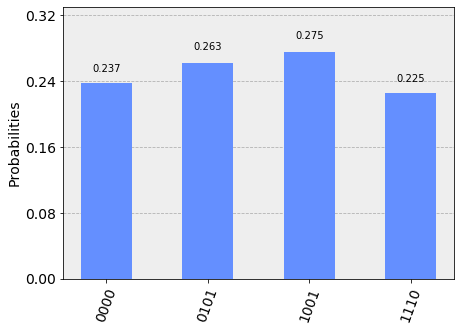
Result showed four state with almost same probability (25%).
0 + 0 = 0, 0 + 1 = 1, 1+0=1, 1+1 = 10(2)
I got more unbalanced data when I run code with low shots.
It is difficult for me to make the quantum circuit for complicated problem.
But technology goes very fast. Quantum computer is used for drug discovery in the feature. So I need to keep my eyes open.
Today’s code is uploaded following URL.
https://nbviewer.jupyter.org/github/iwatobipen/quantum_computing/blob/master/basics_sum.ipynb